Introduction:
Converting a string to a date is a common task in application development. Whether you are working with user input, data files, or API responses, understanding how to correctly parse and format dates is essential. In this blog post, we’ll walk you through how to convert a string to a date in both Java and Kotlin, using modern approaches and best practices.
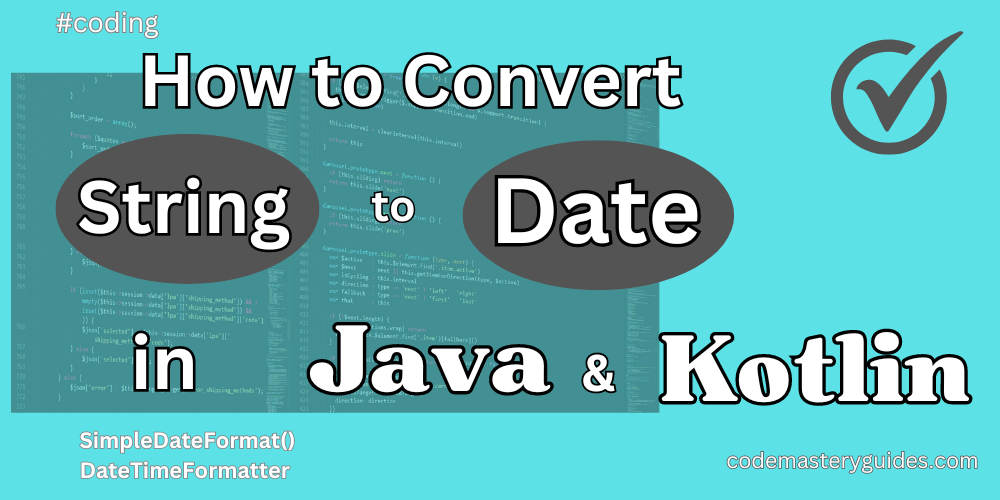
Converting a String to a Date in Java
In Java, the most common way to convert a string to a date is by using the SimpleDateFormat
class. Here’s how you can do it:
Using SimpleDateFormat
:
public class Main { public static void main(String[] args) { String dateString = "2024-05-01"; SimpleDateFormat formatter = new SimpleDateFormat("yyyy-MM-dd"); try { Date date = formatter.parse(dateString); System.out.println("Parsed Date: " + date); } catch (ParseException e) { System.out.println("Error parsing date: " + e.getMessage()); } } }
In the above example, we specify the desired date format ("yyyy-MM-dd"
) and then use the parse()
method of SimpleDateFormat
to convert the string to a Date
object.
Converting a String to a Date in Kotlin
In Kotlin, you can use the standard library’s LocalDate
or LocalDateTime
classes from the java.time
package for date parsing. These classes offer modern and thread-safe methods for working with dates.
Using LocalDate
:
fun main() { val dateString = "2024-05-01" val formatter = DateTimeFormatter.ofPattern("yyyy-MM-dd") val date = LocalDate.parse(dateString, formatter) println("Parsed Date: $date") }
In the above example, we use DateTimeFormatter
with the pattern "yyyy-MM-dd"
to parse the string and convert it to a LocalDate
object.
Using LocalDateTime
:
fun main() { val dateTimeString = "2024-05-01T12:30:45" val formatter = DateTimeFormatter.ISO_DATE_TIME val dateTime = LocalDateTime.parse(dateTimeString, formatter) println("Parsed DateTime: $dateTime") }
In this example, we use the predefined formatter ISO_DATE_TIME
to parse a string representing a date and time.
Conclusion:
Converting a string to a date in both Java and Kotlin is a straightforward process thanks to the built-in classes and libraries available in these languages. By using classes like SimpleDateFormat
in Java or LocalDate
and LocalDateTime
in Kotlin, you can easily parse and manipulate date strings in your applications.
We hope this guide helps you effectively convert strings to dates in your projects. Let us know your thoughts or experiences with date parsing in the comments below.