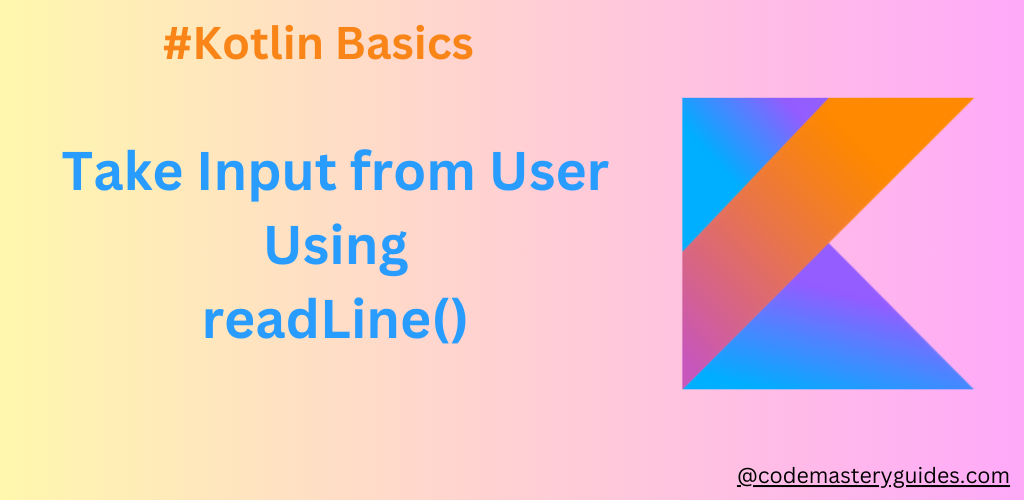
When coding in Kotlin and seeking user input via the console, you have various methods at your disposal to accomplish this task. Keep in mind that Kotlin, being a versatile programming language, provides multiple approaches to facilitate user input in the console.
Input with readLine()
This is the simplest method to obtain input from the Kotlin Console. By using ‘readLine()'
, you can easily capture user input as a String. Save it in a variable, and you’re ready to use it anywhere in your code.
print("Enter Your Name: ")
val userName = readLine()
println("Hello $userName !")
While straightforward and user-friendly, this method encounters challenges when dealing with type conversions.
Type Conversion and Safe Casting
If you want to use readLine() with type conversion and safe casting, follow this method:
print("Enter Your Age: ")
val age = readLine()?.toIntOrNull()
if(age != null){
println("Your age is $age years")
}else{
println("Please Enter valid input for age")
}
In the example above, we convert user input to an integer or null. If the user enters a number, they receive their age; otherwise, an error message is displayed.
Using Scanner for more advanced input
For null-safe and advanced input handling, consider using the Scanner
class. This class auto-casts to any data type, eliminating the need for null checking and manual type conversion.
val scanner = Scanner(System.`in`)
print("Enter Your Age: ")
val age = scanner.nextInt()
println("Your age is $age years")
In the examples above, we also learned how to showcase text in console-based applications.
Conclusion:
To collect user input in Kotlin, you can utilize the readLine()
method for obtaining string input. It also allows for conversion to various data types, including integers. For a safer, null-aware, and auto-casting approach, the Scanner
class is recommended for more advanced input scenarios. It’s important to note that this method is primarily applicable when working with console-based IO. If you’re developing Android applications, Android provides convenient tools like EditText for user input and TextView for displaying output. These components facilitate seamless input and output operations within your Android-based applications.
Click Here for more Kotlin Basics Contents
For more details about Kotlin Basic Inputs, Click Here