Centering text is a common requirement in Android app development, and Kotlin offers several methods to achieve this. In this blog post, we’ll explore different approaches to center text in your Android application.
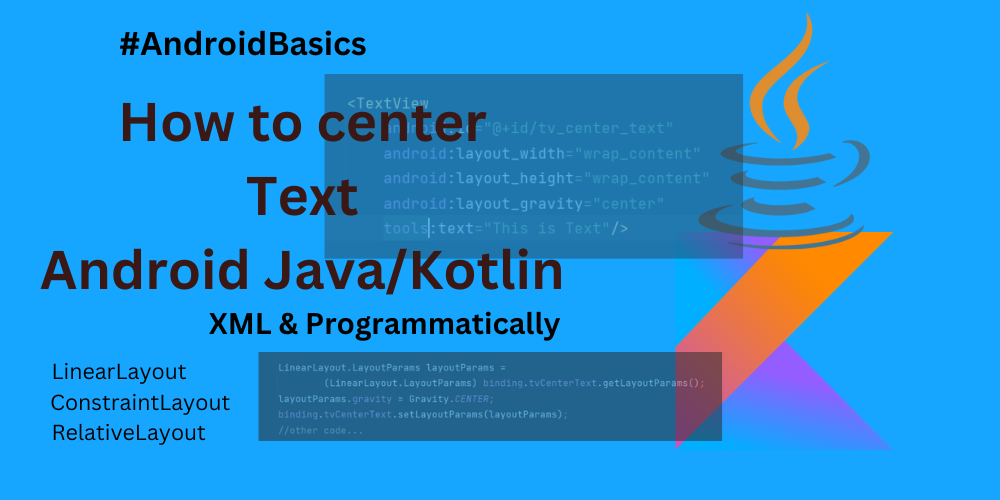
Click Here to learn more about Kotlin Programing language.
Using XML Layouts:
Centering text in Android becomes simple with this simple method, forming the core method. As we explore the various available layout groups, we’ll discover specific techniques crafted to center text in each distinctive layout scenario.
<TextView
android:id="@+id/tv_center_text"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="This is Text..."
android:gravity="center"/>
In the provided code snippet, the android:gravity="center"
attribute is employed to centrally align the text within the TextView. This attribute effectively centers the text within the TextView’s boundaries. This approach proves particularly handy when you set the width as match_parent
or when dealing with multiline text.
Using LinearLayout
If you are using LinearLayout, you’ll center text or other elements using the following method. Make sure you are using layout_width as wrap_content.
<TextView
android:id="@+id/tv_center_text"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="This is Text..."
android:layout_gravity="center"
/>
Using ConstraintLayout:
This layout serves as an excellent alternative to RelativeLayout, particularly when dealing with relationships to other layouts or parent elements. It allows for easy configuration of both horizontal and vertical centering of text.
<TextView
android:id="@+id/tv_center_text"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintTop_toTopOf="parent"
app:layout_constraintBottom_toBottomOf="parent"
android:text="This is Text"/>
In the provided code snippet, we achieve both vertical and horizontal text centering. Should you prefer to center the text in only one direction, feel free to tailor the code accordingly to meet your specific requirements.
Using RelativeLayout:
If you prefer using RelativeLayout, you can use the layout_centerInParent
attribute.
<TextView
android:id="@+id/tv_center_text"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_centerInParent="true"
android:text="This is Text"/>
To center your text either horizontally or vertically, simply utilize android:layout_centerHorizontal="true"
for horizontal alignment and android:layout_centerVertical="true"
for vertical alignment.
Programmatically Centering Text:
If you wish to programmatically center text in Android using Java or Kotlin, you can achieve it with the following code snippet.
Kotlin:
binding.tvCenterText.gravity = Gravity.CENTER
Java:
binding.tvCenterText.setGravity(Gravity.CENTER);
Using LinearLayout:
We will use LinearLayout.LayoutParams to get layout parameters of LinearLayout.
Kotlin:
val layoutParams = binding.tvCenterText.layoutParams as LinearLayout.LayoutParams
layoutParams.gravity = Gravity.CENTER
binding.tvCenterText.layoutParams = layoutParams
Java:
LinearLayout.LayoutParams layoutParams =
(LinearLayout.LayoutParams) binding.tvCenterText.getLayoutParams();
layoutParams.gravity = Gravity.CENTER;
binding.tvCenterText.setLayoutParams(layoutParams);
Using ConstraintLayout:
We will also use LayoutParams to use ConstraintLayout parameters.
val layoutParams = binding.tvCenterText.layoutParams as ConstraintLayout.LayoutParams
layoutParams.startToStart = binding.root.id
layoutParams.endToEnd = binding.root.id
layoutParams.topToTop = binding.root.id
layoutParams.bottomToBottom = binding.root.id
binding.tvCenterText.layoutParams = layoutParams
Choose the method that best suits your app’s layout structure and your personal preferences. These approaches provide flexibility in achieving a centered text in various scenarios within your Android application.
Click Here to Learn more about TextView features.