Introduction:
In Kotlin, a suspend function is designed to be paused and later resumed. This unique feature allows developers to write asynchronous code sequentially, significantly enhancing readability and maintainability when compared to conventional callback-based approaches.
Suspend functions often work with coroutines, lightweight threads that offer a structured approach for handling asynchronous tasks. This ensures smooth and responsive user experiences by preventing the main thread from getting blocked during these operations. Let’s learn a step-by-step guide, on how to use the suspend function in Kotlin.
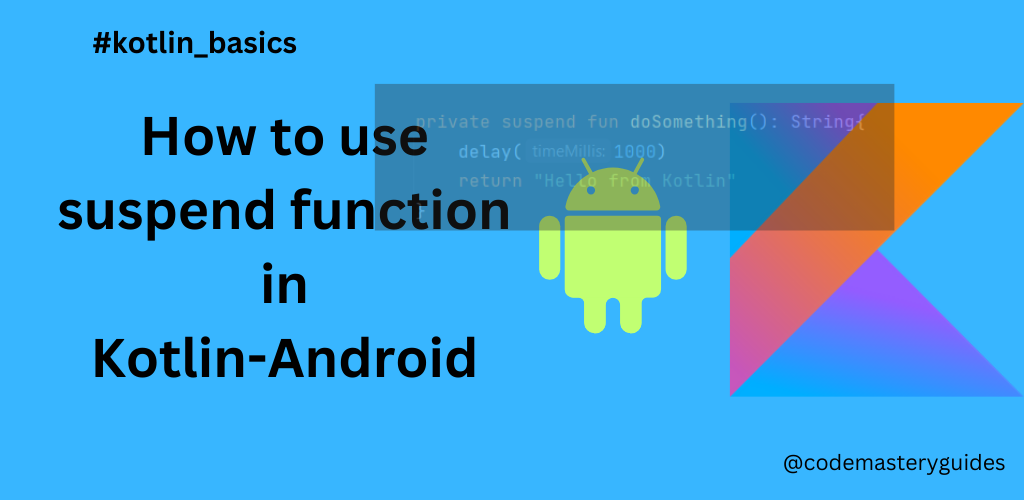
Step 1: Add Coroutines in Gradle:
To begin, integrate the coroutines plugin into your Android Gradle setup. Open the App Level build.gradle file, and in the dependencies section, include the following coroutines plugin.
dependencies {
implementation 'org.jetbrains.kotlinx:kotlinx-coroutines-android:1.3.9'
}
Step 2: Create Suspend function:
Next, define a new function as shown below. This function introduces a one-second delay in the process and then returns the string “Hello from Kotlin.”
suspend fun doSomething(): String{
delay(1000)
return "Hello from Kotlin"
}
Step 3: Launching Coroutines:
To invoke a suspend function, initiate coroutines using the launch
method. It’s essential to launch coroutines when working with suspend functions; otherwise, an error will occur. Here is an example of launching Coroutines:
CoroutineScope(Dispatchers.IO).launch {
val greetings = doSomething()
Log.i("MyTag", greetings)
}
In the provided code snippet, we obtain the greeting text from the doSomething()
method and then log this information under the tag “Log.”
Conclusion:
Suspend functions and coroutines in Kotlin provide a powerful and concise way to handle asynchronous operations in Android development. By using structured concurrency and adopting best practices, developers can create more readable and maintainable code while ensuring a responsive user interface.
Incorporating suspend functions into your Android projects can lead to cleaner and more efficient code, ultimately improving the overall development experience. Experiment with these concepts in your next Android project and witness the benefits of asynchronous programming with Kotlin.
Click Here to learn more about suspend function in Kotlin.
To read more blogs about Kotlin Programming, Click Here.