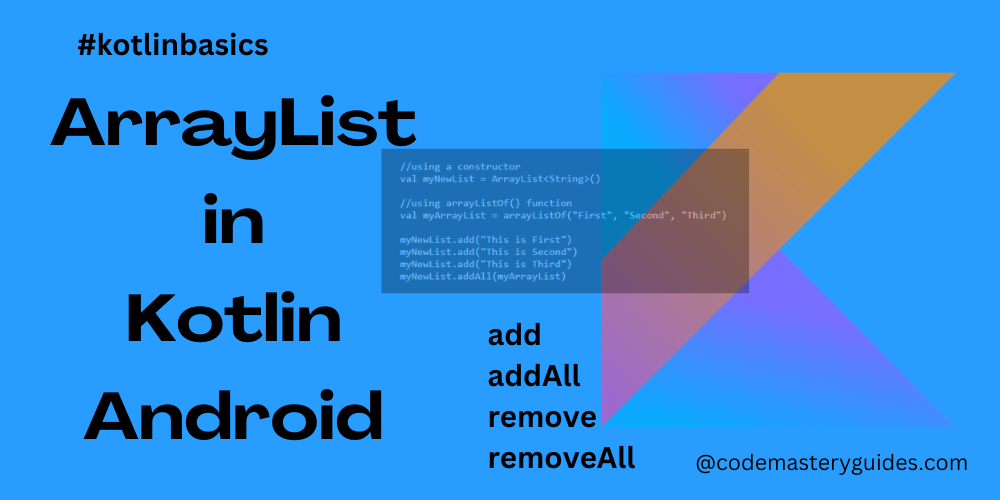
Introduction:
An ArrayList
in Kotlin is a resizable array implementation of the List
interface. Unlike traditional arrays, an ArrayList can dynamically grow or shrink in size, making it a versatile choice for handling collections of data. It is part of the Kotlin Standard Library and provides numerous methods for manipulating and accessing elements efficiently.
Creating an ArrayList:
In Kotlin, crafting an ArrayList is a breeze, thanks to the flexibility offered by either the ArrayList
constructor or the arrayListOf()
function. Let’s explore both options with a concise example:
//using a constructor val myNewList = ArrayList<String>() //using arrayListOf() function val myArrayList = arrayListOf("First", "Second", "Third")
In the preceding example, we employ two distinct approaches. Initially, we fashion an array of strings by leveraging the ArrayList
constructor. Subsequently, we opt for the arrayListOf
method to accomplish the same task. If your objective is to generate an empty array, the first method is your go-to choice. On the other hand, if you intend to initialize an array with predefined elements, the second method is the preferred route.
Adding and Removing Elements:
ArrayList provides methods for adding and removing elements dynamically. Let’s explore some of the commonly used methods:
Adding Elements:
//using a constructor val myNewList = ArrayList<String>() //using arrayListOf() function val myArrayList = arrayListOf("First", "Second", "Third") myNewList.add("This is First") myNewList.add("This is Second") myNewList.add("This is Third") myNewList.addAll(myArrayList)
We employ the add
method to insert a single element into the ArrayList. Should you wish to append multiple elements, the addAll()
method proves invaluable. Simply provide an array of the desired type as an argument. In our illustration, we specifically utilize strings.
Removing Elements:
//remove element with passing an element myNewList.remove("This is First") //remove element with passing element id myNewList.removeAt(2) //remove multiple elements myNewList.removeAll(myArrayList.toSet())
In the initial technique showcased in the above example, the remove
function comes into play, requiring the element to be specified for deletion. However, the second method employs the removeAt
function, necessitating the index of the element to be removed. The third method takes a different approach, utilizing the removal of multiple elements by providing a set or list of elements to be eliminated.
Accessing an Element:
You can retrieve an element from an ArrayList using its index. Kotlin provides a straightforward method for accessing ArrayList elements, as outlined below.
//Accessing an Element val firstElement = myNewList[0]
Accessing All Elements:
If you wish to obtain all elements from an ArrayList, you can utilize the traditional for
loop or the more concise forEach
loop, as illustrated below.
for(value in myNewList){ //Use value as element } myNewList.forEach {value -> //Use value as element }
Immutable List in Kotlin:
While ArrayList provides mutable collections, Kotlin also supports immutable collections. If you want an immutable version of a list, you can use listOf()
instead of ArrayList
:
val mutableList = listOf("First", "Second", "Third", "Fourth")
Conclusion:
In this blog post, we’ve explored the basics of ArrayList in Kotlin, from creation to manipulation and access. ArrayList is a powerful tool for handling dynamic collections of data in a concise and expressive manner, making it a valuable asset in Kotlin programming.
Hi there would you mind letting me know which web host you’re working with?
I’ve loaded your blog in 3 completely different web browsers and I must say this
blog loads a lot faster then most. Can you recommend a good
web hosting provider at a fair price? Kudos, I appreciate it!
i am using hostinger