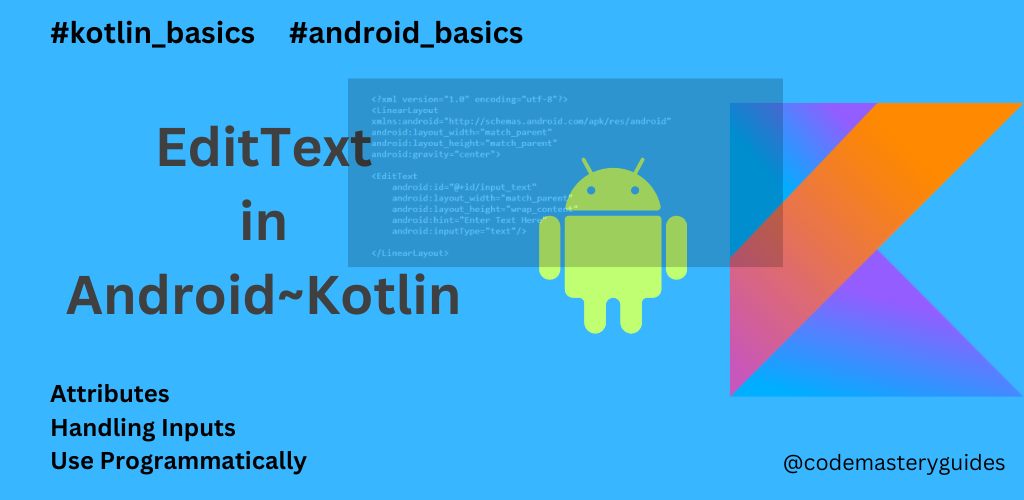
Introduction:
In the world of mobile applications, acquiring user input stands as an essential aspect. Within the domain of Android native programming, EditText emerges as the go-to component for seamlessly gathering input from users.
EditText
, a subclass of the View class in Java and Kotlin programming, plays a vital role in obtaining user input. Whether in XML or programmatically, it offers versatile usage. In this blog, we’ll explore both methods to effectively integrate them into our Android applications.
Use EditText
in the layout file:
In your XML layout file (usually located in the res/layout
directory), you can include an EditText
element to represent the input field. Here’s a simple example:
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="match_parent" android:gravity="center"> <EditText android:id="@+id/input_text" android:layout_width="match_parent" android:layout_height="wrap_content" android:hint="Enter Text Here" android:inputType="text"/> </LinearLayout>
In this example, we’ve added an EditText
with an id
of “input_text.” The hint
attribute provides a placeholder text, and inputType
specifies the type of input (text in this case). We use layout_width
as match_parent
and layout_height
as wrap_content
.
Basic Attributes & Properties:
In this section, we will learn the basic attributes and properties of EditText
.
android:id
: This is the unique identification of every view in the Android layout.
android:layout_width
: This sets the width of the layout. You have the option to use ‘wrap_content,’ ‘match_parent,’ or specify a precise size in dp (density-independent pixels).
android:layout_height
: This sets the height of the layout like above.
android:gravity
: This is used to specify how to align the text inside EditText
. Like, center, start, end, top, bottom, etc.
android:inputType
: Used to set input type for user input. You can set it to, text, number, password, textCapWords, textEmailAddress, textPortalAddress, phone, numberPassword, date, dateTime, time, etc.
android:hint
: Used to display the hint text when EditText
is empty.
android:maxLength
: Used to set the maximum number of characters input.
android:text
: Used to set predefined text in EditText
.
android:textStyle
: Used to define the style of text. You can set it to, bold, italic, or normal.
android:textSize
: Used to set the size of text in EditText. Make sure to use sp
rather than dp
or px
.
android:textColor
: Used to set the color of text.
android:background
: Used to set the background of EditText. Use a drawable file instead of a color for this attribute.
android:backgroundTint
: Used to set the tint of the background for View.
android:drawableStart
: Used to set drawable image at the start of View.
android:drawableEnd
: Used to set drawable image at End of View.
android:drawableTop
: Used to set drawable image at the Top of View.
android:drawableBottom
: Used to set drawable image at Bottom of View.
android:clickable
: Set it to true if you want to set it to clickable and otherwise set it to false.
Use EditText
Programmatically:
Many times, we required to use EditText
Programmatically. In this section, we will learn about how to use EditText
in Programmatically. First, navigate to your Kotlin file and add following code into it:
val editText = EditText(this) val layoutParams = LayoutParams(LayoutParams.MATCH_PARENT, LayoutParams.WRAP_CONTENT) editText.layoutParams = layoutParams editText.hint = "Enter Text Here" editText.typeface = Typeface.DEFAULT_BOLD //Add editText in our root layout binding.root.addView(editText)
We leverage ViewBinding in the provided code. Ensure alignment with your layout file’s root layout by using the corresponding LayoutParams. In this example, we utilize a LinearLayout as the root layout.
Getting Input from EditText
:
For getting input from EditText
, we can use the following method to do so:
binding.button.setOnClickListener { val inputEditText = binding.editText.text.toString() }
You will get editText.text
as Editable, make sure to convert it to String using the toString()
method. To get real-time text changes in EditText
use TextChangedListener
described below:
binding.editText.addTextChangedListener(object : TextWatcher { override fun beforeTextChanged(p0: CharSequence?, p1: Int, p2: Int, p3: Int) { //Add action for before text changed } override fun onTextChanged(p0: CharSequence?, p1: Int, p2: Int, p3: Int) { //Add action on Text changed } override fun afterTextChanged(p0: Editable?) { //Add action after text changed } })
You have to pass TextWatcher
interface with three override functions, beforeTextChanged
, onTextChanged
, and afterTextChanged
.
Input Validations:
There are different ways to check if the text entered by the user in EditText is valid. You can see if the text is valid or ensure that the EditText is not empty before moving on to the next step. Let’s explore some ways to do input validation in EditText.
binding.button.setOnClickListener { val inputEditText = binding.editText.text.toString() if(inputEditText.isEmpty()){ //EditText is empty return@setOnClickListener } if(inputEditText.length > 100 || inputEditText.length < 3){ //EditText input text is too short or too long return@setOnClickListener } }
In the example above, we verify for an empty string as well as a string that is either too short or too long. Feel free to customize the provided code, and you’ll certainly gain additional insights from the experience.
Conclusion:
In conclusion, mastering the usage of EditText in Android Kotlin empowers developers to seamlessly handle user input in their applications. We’ve explored the fundamental concepts, from incorporating EditText in layouts to dynamically interacting with it in Kotlin code. By understanding how to customize appearance, implement input validation, and utilize advanced features, developers can enhance the user experience and create more robust Android applications. As you embark on your journey with EditText, experimentation, and customization are key. Embrace the flexibility that EditText offers, and with each customization, you’ll strengthen your proficiency in Android app development with Kotlin. Happy coding!
Click Here to learn more about EditText.