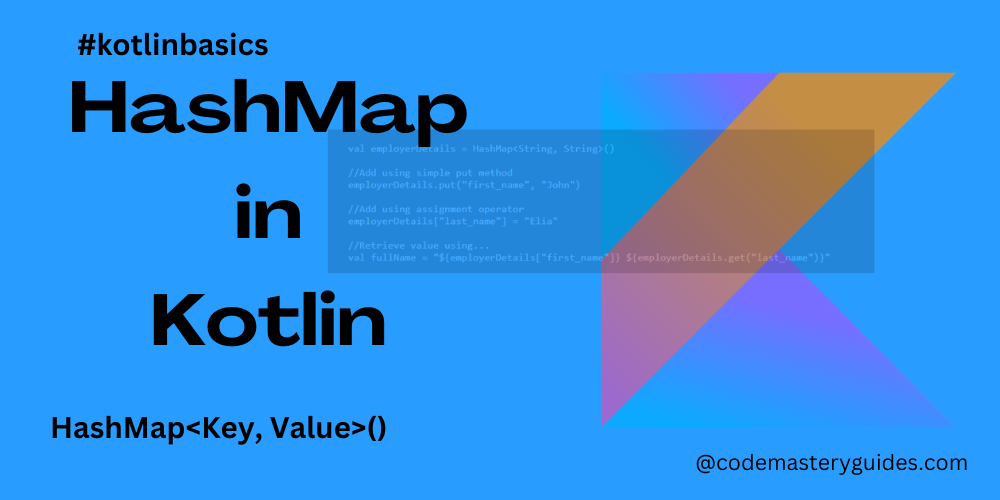
Introduction:
Hashmaps are essential data structures in Kotlin. They provide a way to store and retrieve data efficiently, making them a key component in various applications. In this blog post, we’ll delve into the details of HashMaps, gaining a comprehensive understanding of their inner workings, strengths, and limitations.
A HashMap is a data structure that comprises unique key-value pairs. Each key is distinct and associated with a specific value, allowing efficient retrieval of values based on their corresponding keys. Basic structure of HashMap
is:
HashMap<Key, Value>();
Creating a HashMap:
Creating a HashMap in Kotlin is straightforward. You can declare a HashMap using the HashMap
class or use the mutableMapOf
function for brevity. Here’s an example:
//declare hashMap using HashMap class... val hashMap = HashMap<String, String>() //declare hashMap using mutableMapOf val mutableMap = mutableMapOf<String, String>()
In the code above, we’ve seen two ways to create a HashMap. Both ways are correct and do the same thing.
Adding and Retrieving Values:
Adding and retrieving values from HashMap is also straightforward. Here is an example of how you can add and retrieve values from HashMap.
val employerDetails = HashMap<String, String>() //Add using simple put method employerDetails.put("first_name", "John") //Add using assignment operator employerDetails["last_name"] = "Elia" //Retrieve value using... val fullName = "${employerDetails["first_name"]} ${employerDetails.get("last_name")}"
In the code above, we learned two ways to put in and take out values from a HashMap. The first way uses “put” and “get,” and the second way uses the equals sign (=). If you’re doing stuff in Android Development, it’s suggested to go with the equals sign method instead of using “put” and “get.
Remove Values from HashMap:
Like adding and retrieving, removing value from HashMap
is also important. Here is the method to remove value from HashMap in Kotlin.
employerDetails.remove("first_name")
You can just pass the key to remove the value from HashMap
.
Clear HashMap:
If you wish to completely clear a HashMap and assign values from the beginning, simply use the following code:
employerDetails.clear()
Iterating through a HashMap:
Iterating through a HashMap allows you to perform operations on each key-value pair. Kotlin provides various ways to iterate through a HashMap, and one common approach is to use the for
loop with the entries
property:
for((key, value) in employerDetails){ println("Employer $key is $value") }
Create a List of HashMap:
You can also create a list of HashMaps in Kotlin, just like other objects. Simply declare a mutable list of HashMaps using the following method:
val employerList = mutableListOf(HashMap())
Map vs HashMap:
The HashMap
class in Kotlin implements the Map
interface. However, you can’t use the Map
interface directly in your application. To do so, you must utilize the mapOf
method. It’s important to note that if you opt for this method, it will create a non-mutable map, implying that you won’t be able to add values at runtime.
For scenarios where the dynamic addition of values at runtime is necessary, you’ll need to employ MutableMap
. This allows you to modify the map’s content during the execution of your program, providing the flexibility to insert values as needed.
Conclusion:
Understanding HashMaps in Kotlin is crucial for Android developers. They offer an efficient way to organize and retrieve data in key-value pairs, making them a versatile tool for various scenarios. Whether you’re building a complex Android app or a simple utility, mastering HashMaps will undoubtedly enhance your development skills.