Strings play a essential role in programming, and Kotlin provides powerful and brief ways to manipulate them. In this blog post, we will explore various techniques for concatenating strings in Kotlin, exploring effective and natural ways to improve your coding skills.
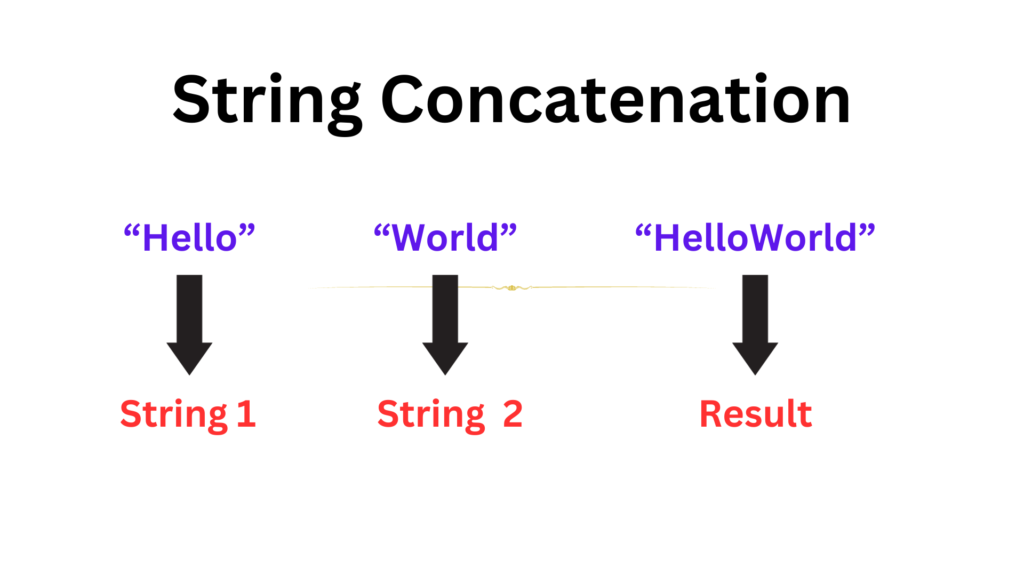
Basic String Concatenation:
In Kotlin, you can concatenate strings using the +
sign, providing a straightforward approach to string concatenation.
val firstName = "Bill"
val lastName = "Gates"
val fullName = firstName + " " + lastName
In the example above, you can concatenate the first name and last name using the +
operator.
String Templates:
Kotlin introduces String Templates, a feature that simplifies string concatenation. With String Templates you can embed variables directly into string, by using $ operator.
val firstName = "Bill"
val lastName = "Gates"
val fullName = "$firstName $lastName"
In the example above, you can perform concatenation using the $
operator. Simply prefix the $
sign to any variable for easy integration. If you wish to utilize additional methods of the object, enclose them within curly brackets following the $ sign. Below is an practical example:
val fullName = "${firstName.uppercase()} ${lastName.uppercase()}"
StringBuilder: Efficient String Concatenation
For frequent String Concatenation, Kotlin provides StringBuilder class. Here is practical example of StringBuilder Class:
val builder = StringBuilder()
builder.append("Bill")
builder.append("Gates")
val finalResult = builder.toString()
In the example above, we instantiate a StringBuilder object named ‘builder’, append two strings to it, and ultimately convert the builder to a string for the final result.
In Android development, where resource productivity and performance are essential, utilizing StringBuilder
appropriately can lead to more responsive and optimized applications.
Concatenating Collections of Strings:
When dealing with lists of strings, Kotlin offers brief ways to concatenate them. To concatenate a list of strings, you can utilize the joinToString
method
val stringList = listOf("One", "Two", "Three", "Four")
stringList.joinToString(separator = "," , prefix = "Numbers: ", postfix = ".")
In the above example, you can concatenate a list of strings using a specified separator, along with optional prefix and postfix. Result of above code will be:
Numbers: One,Two,Three,Four.
Conclusion:
Mastering string concatenation in Kotlin is very important skill for any developer. In this blog post, we’ve covered basic concatenation, string templates, the use of StringBuilder
for efficiency, and concatenating collections of strings. Armed with these techniques, you’ll be well-equipped to handle diverse string manipulation tasks in your Kotlin projects.
Explore more articles on Android Kotlin by clicking here.
Click here to learn more about using strings in Kotlin.