Introduction:
In Android apps, calculating percentages is a common task, used for things like progress bars or score computations. This tutorial will guide you on efficiently performing percentage calculations in Android using Kotlin. We will follow a step-by-step guide to calculate the percentage.
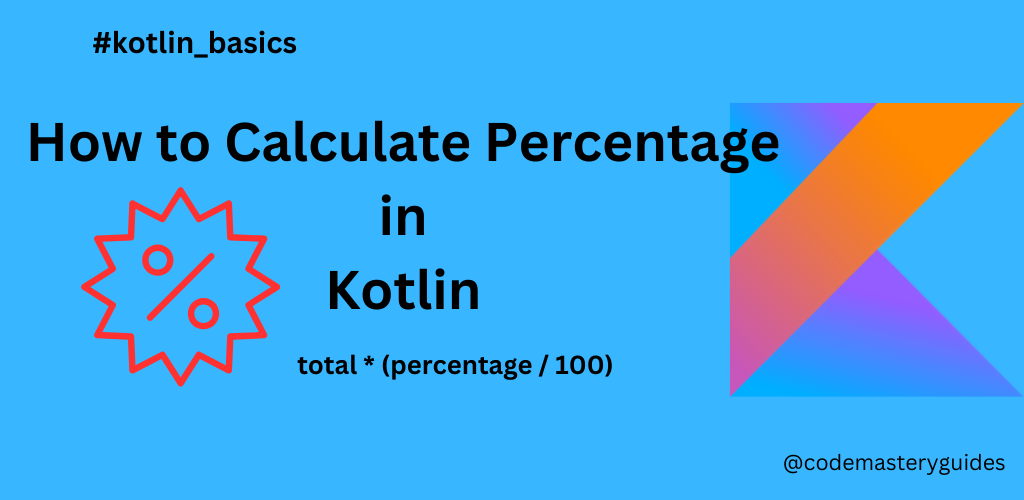
Step 1: Understand Percentage:
Before writing any code, let’s first understand how percentage works. A percentage represents a part of a whole, where the whole is considered as 100%. To find a percentage, you determine a fraction of the total and express it relative to 100.
Step 2: Setting Up your Android Project:
Begin by creating a new project: navigate to File => New => New Project, choose your preferred settings, and click Finish. Make sure you have the required dependencies installed and a fundamental understanding of Kotlin programming.
Step 3: Design UI
Design a simple user interface with input fields for the total and the percentage, and a button to trigger the calculation.
<androidx.appcompat.widget.LinearLayoutCompat
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
android:gravity="center"
tools:context=".MainActivity3">
<EditText
android:id="@+id/et_per_total"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:hint="Enter Total Here"
android:inputType="number"
android:layout_margin="10dp"/>
<EditText
android:id="@+id/et_per_percentage"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:inputType="number"
android:hint="Enter Percentage Here"
android:layout_margin="10dp"/>
<Button
android:id="@+id/btn_calculate_per"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_margin="10dp"
android:text="Calculate"/>
</androidx.appcompat.widget.LinearLayoutCompat>
To ensure numeric input for both EditTexts, set the inputType
attribute to ‘number’.
Step 4: Implementing the Calculation Logic:
Now, let’s implement the percentage calculation logic in the Kotlin class.
private fun calculatePercentage(): Double{
val total = binding.etPerTotal.text.toString().toDouble()
val percent = binding.etPerPercentage.text.toString().toDouble()
return total * (percent / 100)
}
The provided code will generate the result as a double
. For instance, when calculating 20% of 200, the app will return a decimal value of 40.
200 * (20 / 100) = 40
Click Here to learn more about Arithmetic Operators in Kotlin.
Final Code:
class MainActivity : AppCompatActivity() {
private lateinit var binding: ActivityMain3Binding
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
binding = ActivityMainBinding.inflate(layoutInflater)
setContentView(binding.root)
binding.btnCalculatePer.setOnClickListener {
val per = calculatePercentage()
//use percentage where you require...
}
}
private fun calculatePercentage(): Double{
val total = binding.etPerTotal.text.toString().toDouble()
val percent = binding.etPerPercentage.text.toString().toDouble()
return total * (percent / 100)
}
}
Conclusion:
Congratulations! You’ve now implemented a simple Android app in Kotlin to calculate percentages. This example covers the basics, but you can further enhance and customize it based on your specific requirements.
Feel free to add error handling, improve the user interface, or integrate the calculation into a more extensive application. Happy coding!
Click Here to read more articles about Kotlin Programming.