Introduction:
Mathematics plays a crucial role in programming, and one fundamental mathematical operation often encountered is exponentiation, or raising a number to a certain power. In this blog post, we will delve into the concept of power and explore various ways to calculate it using the Kotlin programming language.
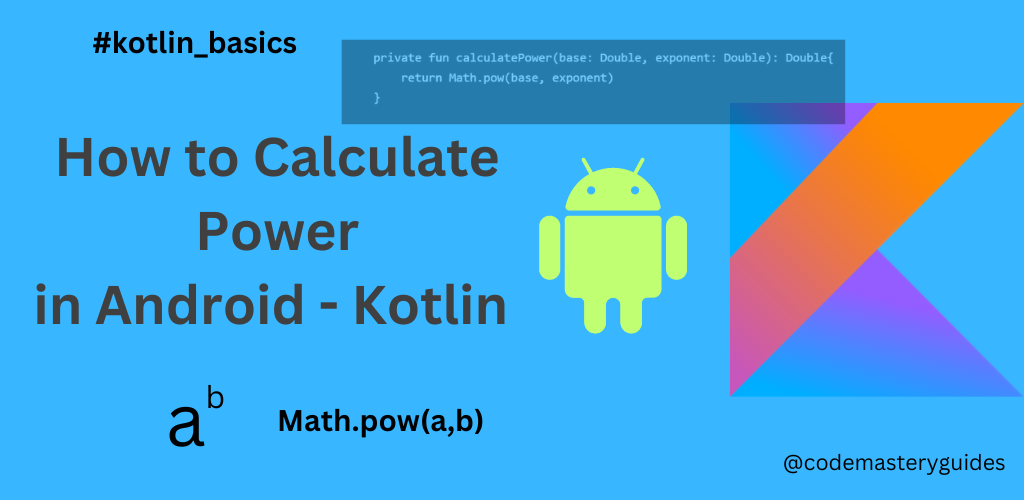
What is Power in Mathematics:
Power in mathematics refers to the operation of raising a base to an exponent. The expression ab involves two components: ‘a’ as the base and ‘b’ as the exponent. In simple terms, the result is obtained by multiplying the base ‘a’ by itself ‘b’ times.
Calculate Power using Loop:
This traditional approach involves utilizing a loop, either ‘for’ or ‘while,’ where we iterate ‘b’ times in the case of ab. During each iteration, the result is multiplied by ‘a.’ However, this method has limitations, particularly with large values of ‘b.’ As ‘b’ increases, it leads to slower execution and, consequently, a sluggish application process.
private fun calculatePower(base: Double, exponent: Double): Double{ var result = 1.0 for(i in 1..exponent.toInt()){ result *= base } return result }
This method is effective only when the exponent is a natural number. If the exponent is less than 0, it fails to produce the correct result.
Using Math.pow function:
Kotlin provides a built-in function, Math.pow
, for power calculations. This function takes two parameters: the base and the exponent, and returns the result as a double.
private fun calculatePower(base: Double, exponent: Double): Double{ return Math.pow(base, exponent) }
The Math.pow
function simplifies power calculations and is suitable for general use. However, Kotlin provides a built-in pow
function for doubles, allowing for convenient exponentiation. This function takes two parameters: the base and the exponent. It simplifies power calculations, making it straightforward to raise a number to a given power in Kotlin.
private fun calculatePower(base: Double, exponent: Double): Double{ return base.pow(exponent) }
Conclusion:
In this blog post, we explored different approaches to calculating power in Kotlin. From the traditional loop-based method to leveraging the built-in Math.pow
function. As you delve into mathematical operations in your Kotlin projects, consider these methods to enhance your programming skills.
Click Here to learn more about Math in Kotlin.