Introduction:
In Android development, Kotlin and Java are crucial languages. Often, we need to call Kotlin methods from Java and vice versa. This blog guides you on how to smoothly achieve this bidirectional communication, enabling seamless integration of Kotlin and Java in your Android projects.
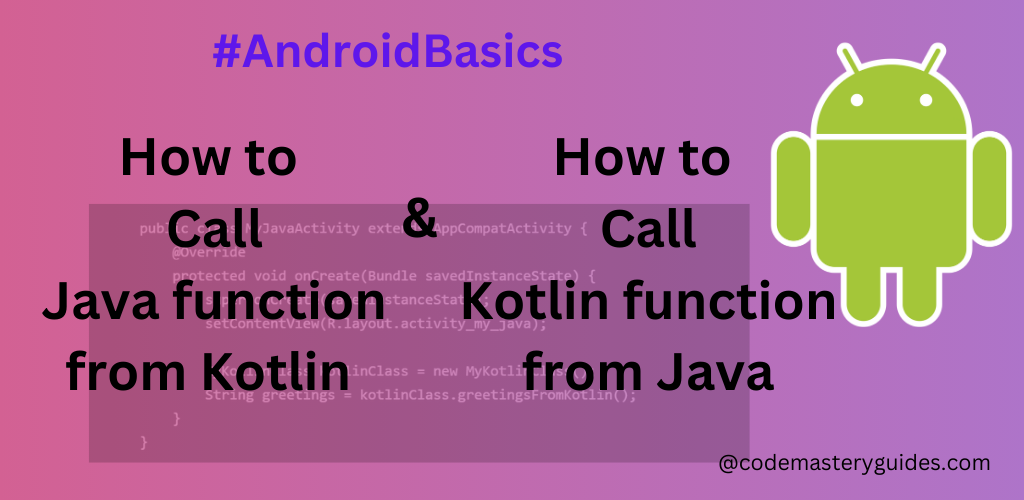
Call Kotlin function from Java:
Step 1: Create a new Activity:
First, create a new Activity in Java and Kotlin. Make sure you have a proper understanding of how to create a new Activity. If you want to learn how to create a new Activity, Click Here to learn.
Step 2: Create a function in Kotlin:
Next, establish functions in Kotlin, and subsequently, invoke these functions from Java.
class MyKotlinClass {
fun greetingsFromKotlin(): String{
return "Hello Kotlin!"
}
}
Step 3: Call the function from Java Activity:
Now, let’s call the function from a Java Activity or Class. Start by creating an object of the MyKotlinClass class and proceed to invoke it as outlined below.
public class MyJavaActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_my_java);
MyKotlinClass kotlinClass = new MyKotlinClass();
String greetings = kotlinClass.greetingsFromKotlin();
}
}
Call Java function from Kotlin:
Now, let’s delve into calling Java functions from Kotlin. Initially, create a Kotlin class and define a new function. Following that, establish a Java class or Activity, and invoke the Kotlin class object to call the newly created function. Let’s illustrate this process through code.
Step 1: Create a new Java function:
Create a new Java Class and invoke a new function as described below:
public class MyJavaClass {
public String greetingFromJava(){
return "Hello Java!";
}
}
Step 2: Call the function from Kotlin Activity:
Call above function from Kotlin Activity or class using following code:
class MyKotlinActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_my_kotlin)
val javaClass = MyJavaClass()
val greetings = javaClass.greetingFromJava()
}
}
In the provided code snippet, we declare a MyJavaClass object, invoke the function, and retrieve the returned value.
Conclusion:
In conclusion, mastering the art of calling Kotlin functions from Java and vice versa proves to be an invaluable skill for developers navigating the dynamic landscape of mixed-language Android projects. This seamless interoperability between Kotlin and Java allows for the gradual adoption of Kotlin’s modern features while maintaining compatibility with existing Java codebases. Armed with the knowledge shared in this guide, developers can confidently bridge the gap between these two languages, fostering a more cohesive and efficient development process. Whether migrating from Java to Kotlin or simply integrating the two languages in a project, the bidirectional communication techniques outlined herein empower developers to harness the strengths of both Kotlin and Java, unlocking new possibilities in Android development.
Learn More about Java Programming language using Android, Click Here
Learn More about Kotlin Programming language using Android, Click Here