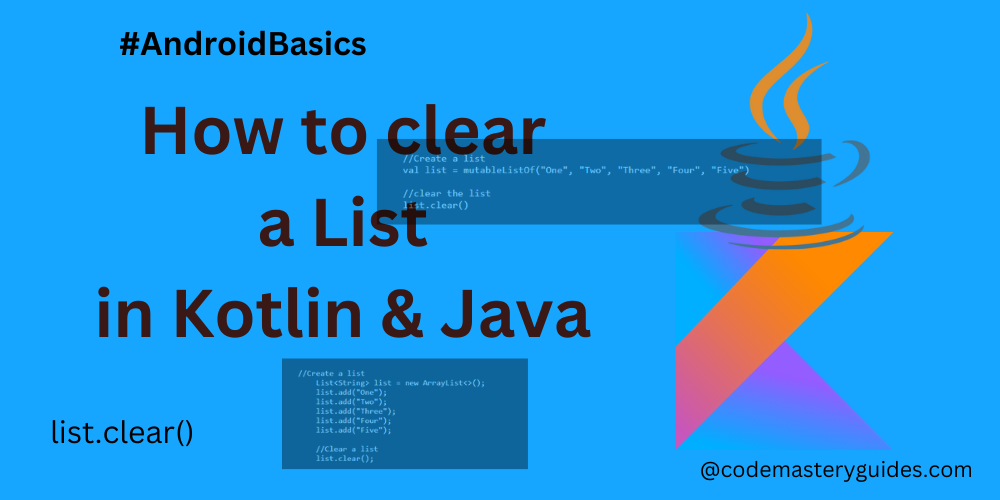
Introduction:
Lists are a fundamental data structure in programming, allowing us to store and manipulate collections of elements. Many times, we need to clear our list and add some more elements to it. In this blog, we will learn about how to clear a list and remove all elements from an array list in Java and Kotlin.
Clear a list in Kotlin using clear()
method:
In Kotlin, clearing a list is a straightforward process. Kotlin provides the clear()
method, which removes all elements from the list. Below is an example of how to clear a list in Kotlin.
//Create a list val list = mutableListOf("One", "Two", "Three", "Four", "Five") //clear the list list.clear()
In the provided code snippet, we initialize a list of strings containing five elements. To efficiently remove all elements from this list, we employ the clear()
method. This method acts as a swift and concise solution, effectively erasing all existing elements, and leaving the list in an empty state.
Clear a list in Java using clear()
method:
In Java, the process is slightly different. The clear()
method is also used, but it is called on an instance of the List
interface. Here’s an example:
//Create a list List<String> list = new ArrayList<>(); list.add("One"); list.add("Two"); list.add("Three"); list.add("Four"); list.add("Five"); //Clear a list list.clear();
In Java, the ArrayList
class is used as an implementation of the List
interface. The clear()
method removes all elements from the list, making it empty.
Conclusion:
Clearing a list is a common operation in programming, and understanding how to do it in both Kotlin and Java is essential. Whether you are working with Kotlin’s concise syntax or Java’s more explicit approach, the clear()
method is your go-to solution for efficiently emptying a list. Incorporate these techniques into your code to manage and manipulate lists effectively.