Introduction:
When working with collections in Kotlin and Java, you might encounter situations where you need to convert a List into an ArrayList. This conversion can provide you with greater flexibility and access to various methods available in the ArrayList class. In this blog post, we’ll explore how to convert a List to an ArrayList in both Kotlin and Java using modern approaches and standard libraries.
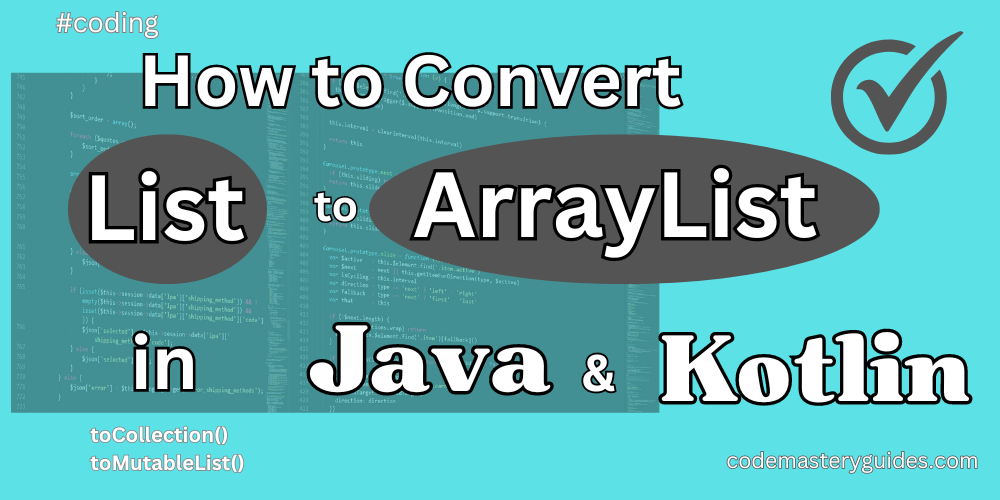
What Is an ArrayList?
An ArrayList
is a dynamic array implementation that allows you to store elements and modify the size as needed. It offers various methods for manipulating the data it holds, such as adding, removing, or updating elements.
Converting a List to ArrayList in Java
In Java, you can convert a List
to an ArrayList
using various methods. Let’s explore some of them:
Method 1: Using Constructor:
You can use the constructor of ArrayList
to convert a List
to an ArrayList
directly:
public class Main { public static void main(String[] args) { Listlist = List.of("Apple", "Banana", "Cherry"); ArrayList arrayList = new ArrayList<>(list); System.out.println(arrayList); } }
Method 2: Using the Stream API (Java 8+)
If you’re working with Java 8 or later, you can use the Stream
API to convert a List
to an ArrayList
:
public class Main { public static void main(String[] args) { Listlist = List.of("Apple", "Banana", "Cherry"); ArrayList arrayList = list.stream().collect(Collectors.toCollection(ArrayList::new)); System.out.println(arrayList); } }
Converting a List to an ArrayList in Kotlin
In Kotlin, you can convert a List
to an ArrayList
using various methods provided by the Kotlin standard library.
Method 1: Using toCollection
Kotlin’s standard library provides the toCollection
function to convert a List
to an ArrayList
:
fun main() { val list = listOf("Apple", "Banana", "Cherry") val arrayList = list.toCollection(ArrayList()) println(arrayList) }
Method 2: Using toMutableList
You can also use toMutableList
to convert a List
to a mutable list (ArrayList
):
fun main() { val list = listOf("Apple", "Banana", "Cherry") val mutableList = list.toMutableList() println(mutableList) }
Conclusion:
Converting a List
to an ArrayList
in both Kotlin and Java is straightforward, thanks to the powerful standard libraries and modern features available in these languages. Whether you’re using Java’s Stream
API or Kotlin’s collection functions, you can easily achieve the desired conversion. These methods offer convenience and flexibility when working with collections in your projects.
Happy coding! Let us know your thoughts or experiences with these conversions in the comments below.