Text Formatting is a very important aspect of visualizing text in Android Applications. In this tutorial, we will discuss how to make text bold in TextView in Android using Java and Kotlin. We will follow a step-by-step guide to make text bold in Textview. This guide will focus on Java and Kotlin both.
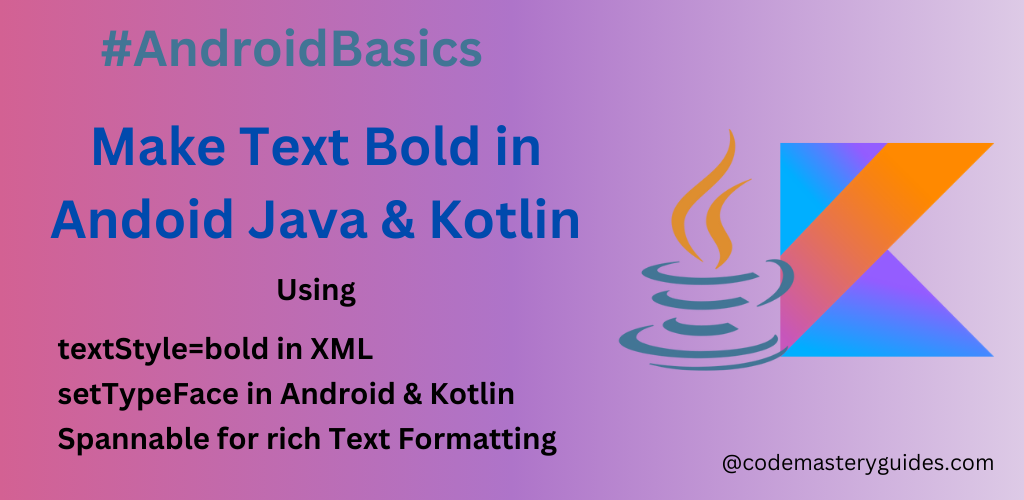
By Using XML:
This is the easiest way to make text bold in a TextView. Open your XML file and add the following TextView tag:
<TextView
android:id="@+id/text_view"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Hello, Code Mastery Guides!"
android:textStyle="bold"
/>
In the code above, we’ve set up a TextView with its id, width, height, and text parameters. To make the text bold in Android TextView, we’ve added a new parameter: textStyle="bold"
. This parameter enhances the visual presentation of the text.
Programmatically make text bold:
Kotlin:
Now, let’s programmatically make the text bold in Android using Kotlin. First, ensure you’re using viewBinding and initialize it in your YourActivity.kt
. Follow the steps below to achieve bold text.
//Use below code in app version of build.gradle file in android
android{
//other code...
buildFeatures{
viewBinding true
}
}
//Use below code in YourActivity.kt
class MainActivity : AppCompatActivity() {
private lateinit var binding: ActivityMainBinding
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
binding = ActivityMainBinding.inflate(layoutInflater)
setContentView(binding.root)
//code to make text bold...
binding.textView.setTypeface(null, Typeface.BOLD)
//other code...
}
In the code snippet above, we’ve employed view binding to declare the textView, although other methods are also available for declaration. The setTypeface
method is then utilized to set the text type, where we pass a null parameter for the Typeface class and specify ‘Bold’ for the type parameter.
Java:
Now, let’s implement the above code in the Java programming language. We’ll also utilize viewBinding in Java as demonstrated earlier. For brevity, we’ll skip the binding call for now.
public class MainActivity2 extends AppCompatActivity {
private ActivityMain2Binding binding;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
binding = ActivityMain2Binding.inflate(getLayoutInflater());
setContentView(binding.getRoot());
//code to make text bold in TextView
binding.textView.setTypeface(null, Typeface.BOLD);
//other code...
}
}
Spannable for rich text formatting:
To make only a specific part of the text bold, you can utilize Spannable in such cases. You can use SpannableString and StyleSpan for it.
val greetings = "Hello"
val spannable = SpannableString(greetings)
val boldSpan = StyleSpan(Typeface.BOLD)
spannable.setSpan(boldSpan, 0, greetings.length, Spannable.SPAN_EXCLUSIVE_EXCLUSIVE)
val builder = StringBuilder()
builder.append(spannable)
val name = "John"
builder.append(name)
binding.textView.setText(spannable, TextView.BufferType.SPANNABLE)
In the preceding code, we employ Spannable to set the typeface to bold for greetings, while the rest of the text remains in normal style.
Conclusion:
In this tutorial, we’ve covered the basics of making text bold TextView
using Kotlin in Android. The process is straightforward and can be done both programmatically and through XML styling. As you delve deeper into Android development, exploring additional text formatting options will empower you to create more engaging user interfaces.
Click Here for more details about TextView formatting.
Click Here to read more blogs related to the Kotlin Programming Language.